How to build a Cypress Automation Framework From Scratch?
- Shyedhu
- Jun 6, 2021
- 3 min read
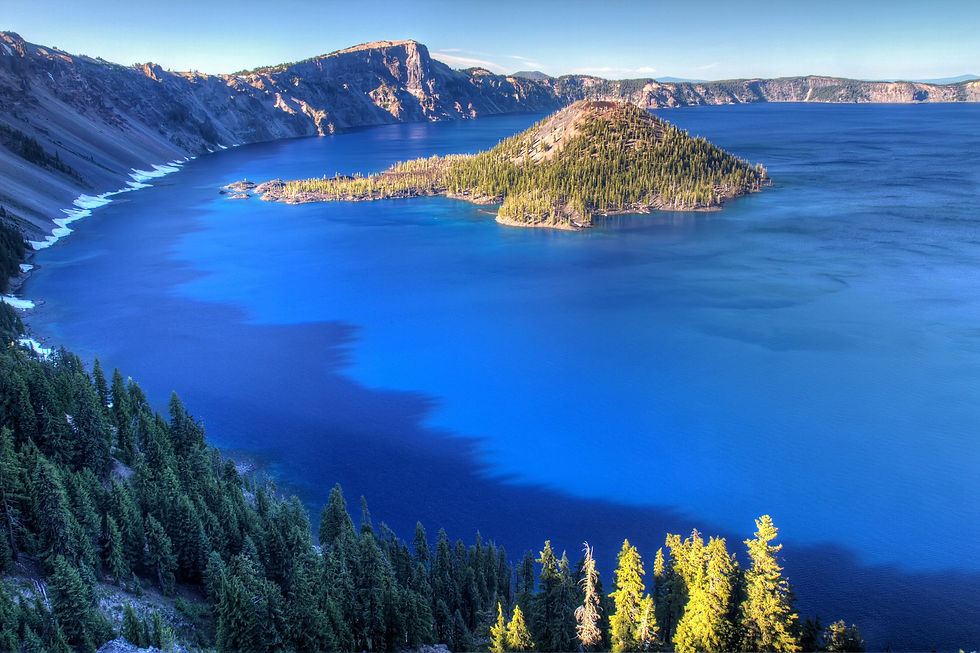
In this blog, I’m going to show you how to build Web Automation Framework from scratch using cypress.io with Javascript.
Prerequisites
- Install Node.js (together with the NPM tool) instructions are available on https://nodejs.org/en/
- Install Docker, instructions are available on https://docs.docker.com/get-docker/
Getting Started with Cypress
Let’s start by creating a folder for our automation framework. In your terminal run the following commands:
mkdir cypress-automation-framework
cd cypress-automation-framework
Now let’s add a package.json file to import all of required dependencies for cypress automation framework
Add the following to package.json file
{
"scripts": {
"cy:open": "./node_modules/.bin/cypress open",
"lint:run": "eslint '**/*.js'",
"lint:fix": "eslint --fix '**/*.js'"
},
"dependencies": {
"cypress": "7.4.0",
"ls": "^0.2.1",
"mocha": "^5.2.0",
"mochawesome": "^4.1.0",
"mochawesome-merge": "^4.0.0",
"rimraf": "^3.0.2",
"yargs": "^15.1.0"
},
"devDependencies": {
"cypress-log-to-output": "^1.0.8",
"eslint": "^7.27.0",
"eslint-config-airbnb-base": "^14.2.1",
"eslint-plugin-import": "^2.23.4"
}
}
Next, Let’s create a few sub folders in root folder
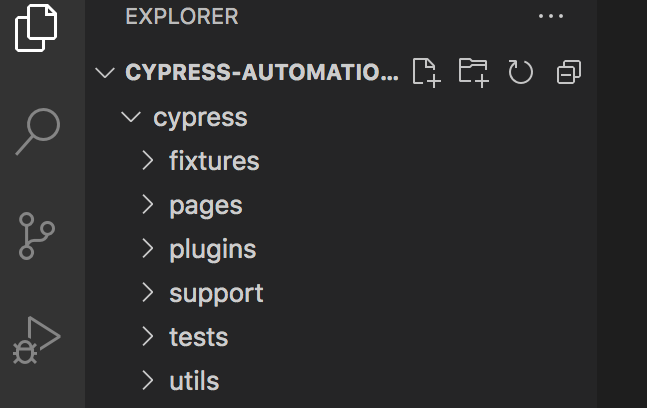
Next, Let’s add cypress.json configuration file in root folder,This JSON file is used to store any configuration values you supply.
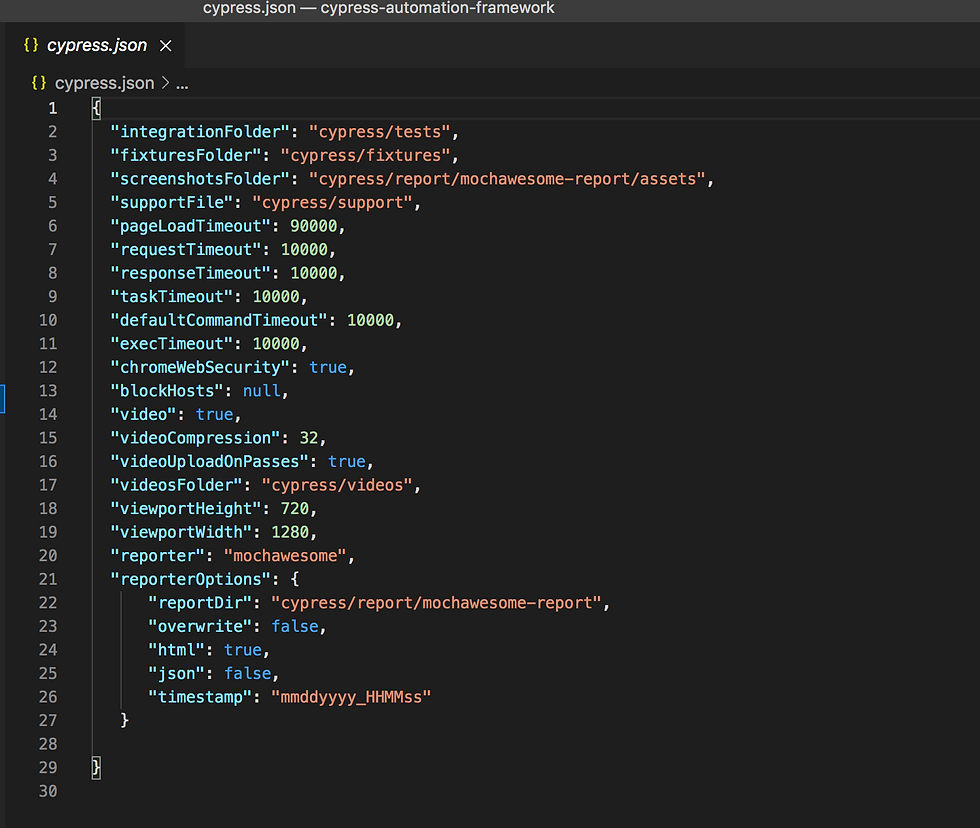
Next, Let’s add Dockerfile file in root folder,This is a simple text file that consists of instructions to build Docker images.
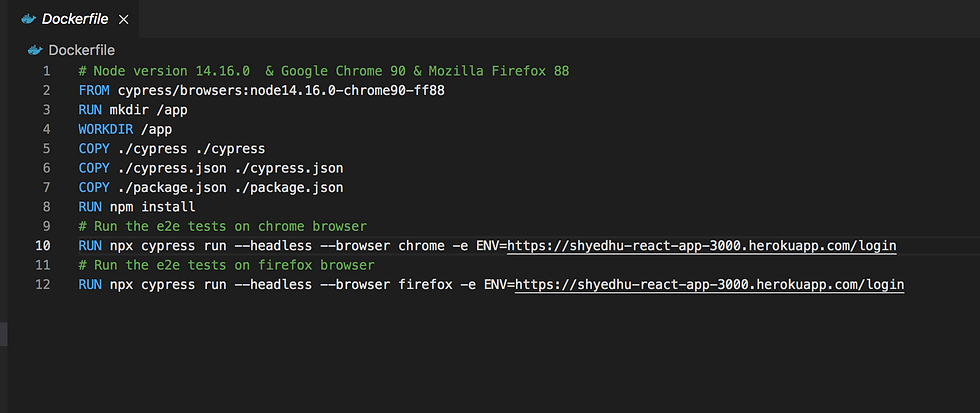
Next, Let’s add Jenkinsfile in root folder,This is a text file that contains the definition of a Jenkins Pipeline and is checked into source control.
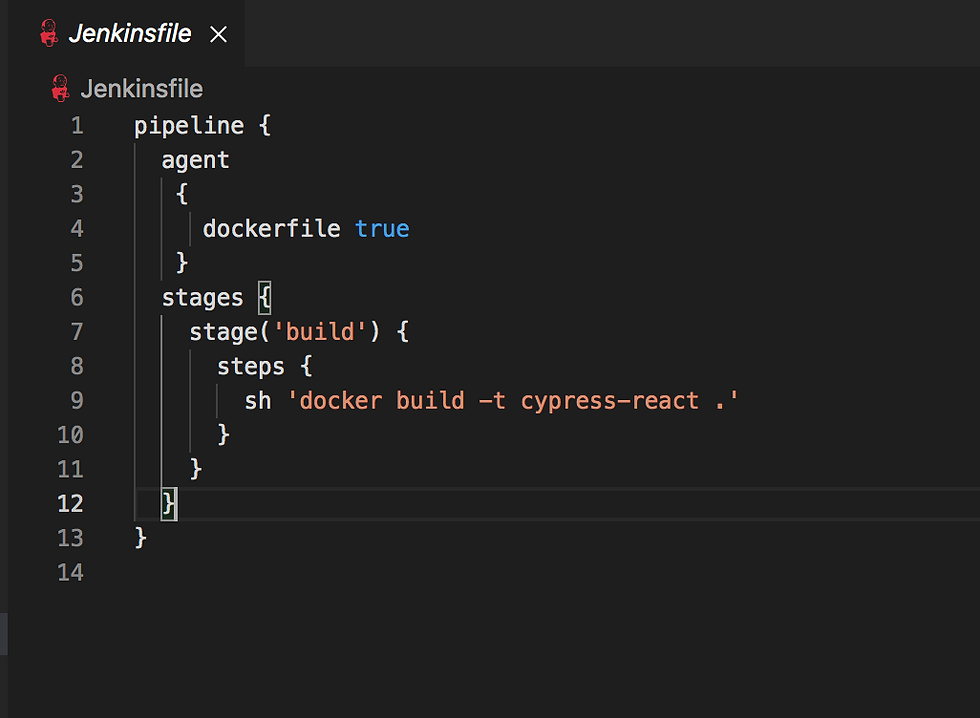
Here's cypress automation framework's project structure
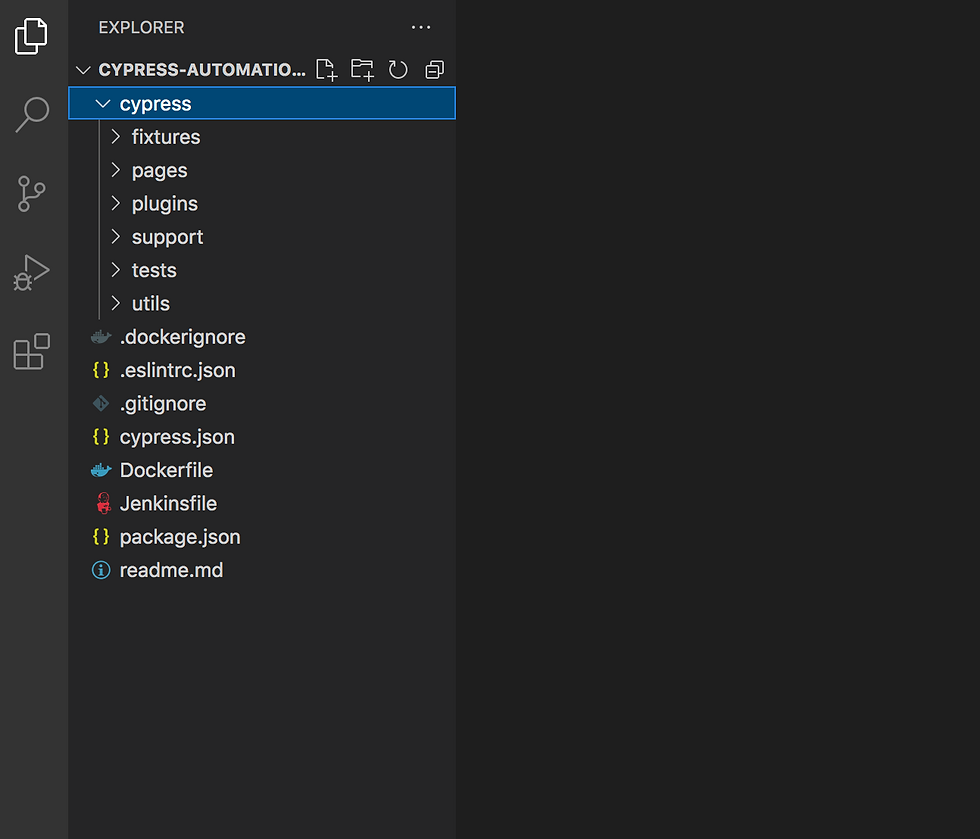
Next , Let's add products.json and users.json files in fixtures folder, This is .json file should be in the fixtures folder as cypress will automatically search for test data inside this folder by default until and unless the path is specified otherwise.

Next , Let's add page-objects files for each react page in pages folder, This file contains Locator strategy for each react page.
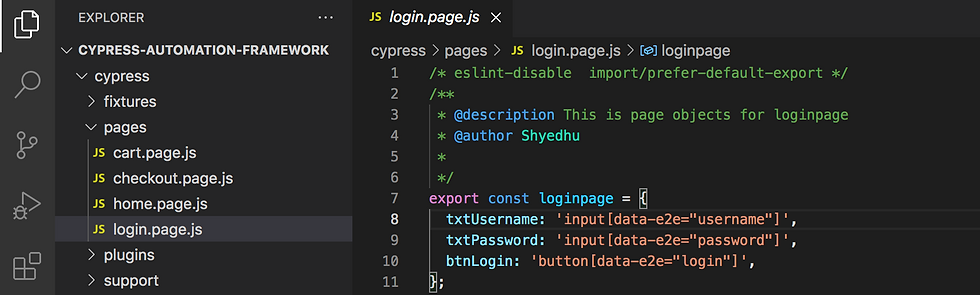
Next , Let's add Custom Commands in support folder. Cypress provides an API for creating a new custom command or overwriting existing commands to change their implementation. The built-in Cypress commands also use the API.

Next , Let's add test files for each test scenarios in tests folder
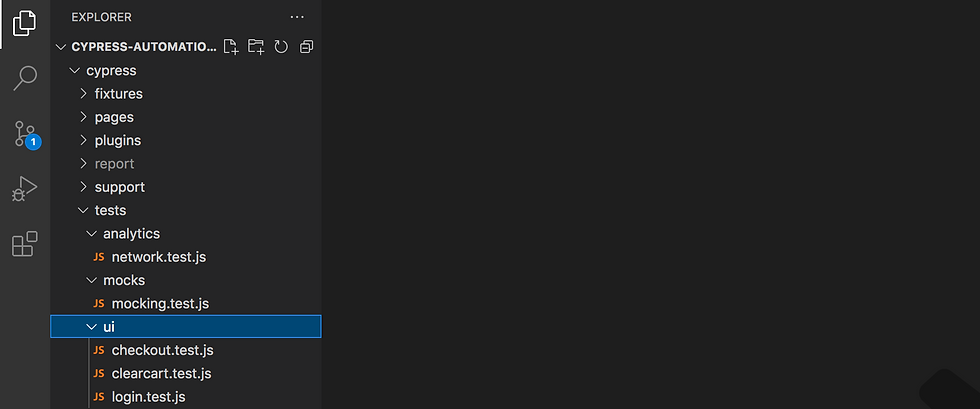
Writing UI Test
Now, add a file name login.test.js under tests folder and add the following statements to it:
/**
* @description Test class to validate the login page
* @author Shyedhu
*
*/
describe('Validate the login page', () => {
beforeEach(() => {
cy.fixture('users.json').as('usersData');
custom.navigateTo(Cypress.env('ENV'));
});
it('test the login page', () => {
cy.get('@usersData').then((usersData) => {
// validate the login
cy.login(usersData.username, usersData.pass);
cy.logout();
});
});
});
This is login test will let users navigate to https://shyedhu-react-app-3000.herokuapp.com/login and then validate the login flow
Next, Let's see the Mock Data Approaches using cypress. Most of the web applications have a backend API that serves data. Setting up the data is essential to write more tests and is often a painful task. Let us explore a different options to set up mock data for testing using cypress.
There are two ways we could be mocking, 1. Inline code Mocking 2. Fixtures
Let’s see mocking data using Fixtures approach which is essentially the same as Inline code Mocking but instead of having the response as JavaScript code we point to a JSON file .
Make sure add following products.json in fixtures folder
[
{
"id": 1,
"name": "Mango",
"image": "mango.png",
"available_quantity": 50,
"price": 7,
"description": "This is Mango"
}
]
we can now instruct cypress to use the above JSON file like so:
cy.fixture('products.json').as('productsData');
// mocking the products api response data
cy.route('GET', 'https://nodes-api-demo.vercel.app/api/products', '@productsData').as('productsapi');
Here's one of mocking test using cypress
/**
* @description Test class to mock products api response data
* @author Shyedhu
*
*/
describe('Validate the macking response', () => {
beforeEach(() => {
cy.fixture('users.json').as('usersData');
cy.fixture('products.json').as('productsData');
cy.server();
// mocking the products api response data
cy.route('GET', 'https://nodes-api-demo.vercel.app/api/products', '@productsData').as('productsapi');
custom.navigateTo(Cypress.env('ENV'));
});
it('test mocking response', () => {
cy.get('@usersData').then((usersData) => {
// validate the login
cy.login(usersData.username, usersData.pass);
cy.wait('@productsapi');
// Assert on XHR
cy.get('@productsapi').then((xhr) => {
// Assert status code
expect(xhr.status).to.eq(200);
// Assert request method
expect(xhr.method).to.eq('GET');
// Assert request url
expect(xhr.url).to.match(/\/products$/);
// Assert response Body
const response = xhr.responseBody;
expect(response[0]).to.have.property('available_quantity', 50);
expect(response[0]).to.have.property('price', 7);
expect(response[0]).to.have.property('description', 'This is Mango');
});
});
});
})
Running e2e tests on locally and docker
First , Navigate into the project root folder and then install all dependencies using following command. This will install all of the necessary packages to get cypress installed on your local machine.
npm install
Running tests on chrome browser
npm run cy:run:chrome
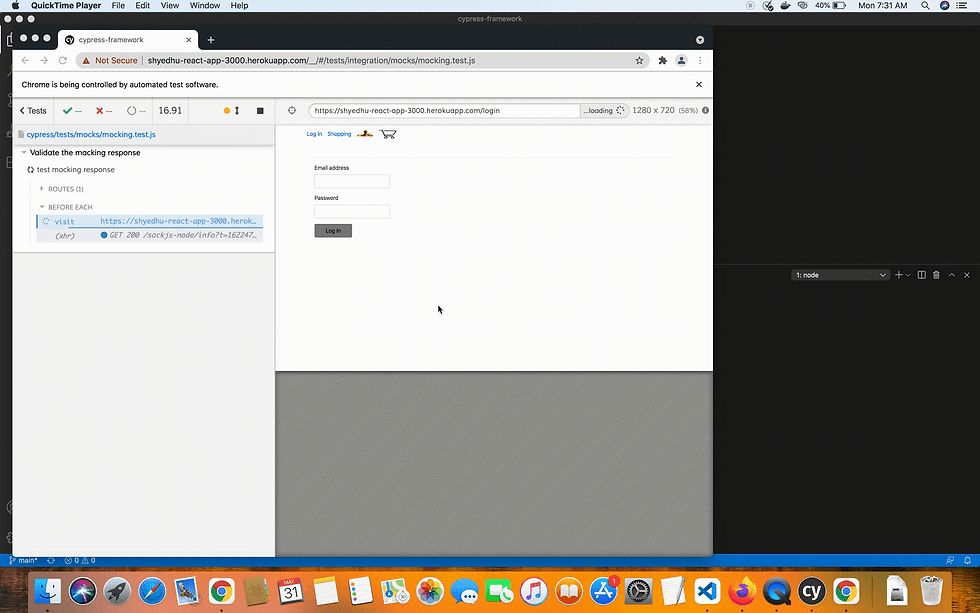
Running tests on firefox browser
npm run cy:run:firefox
Running tests on docker
docker build -t cypress-react .
View the Mochawesome report and Videos
1.In order to view the html report , you will need to navigate to /cypress-automation-framework/cypress/report/mochawesome-report
2. View the videos , you will need to navigate to /cypress-automation-framework/cypress/videos
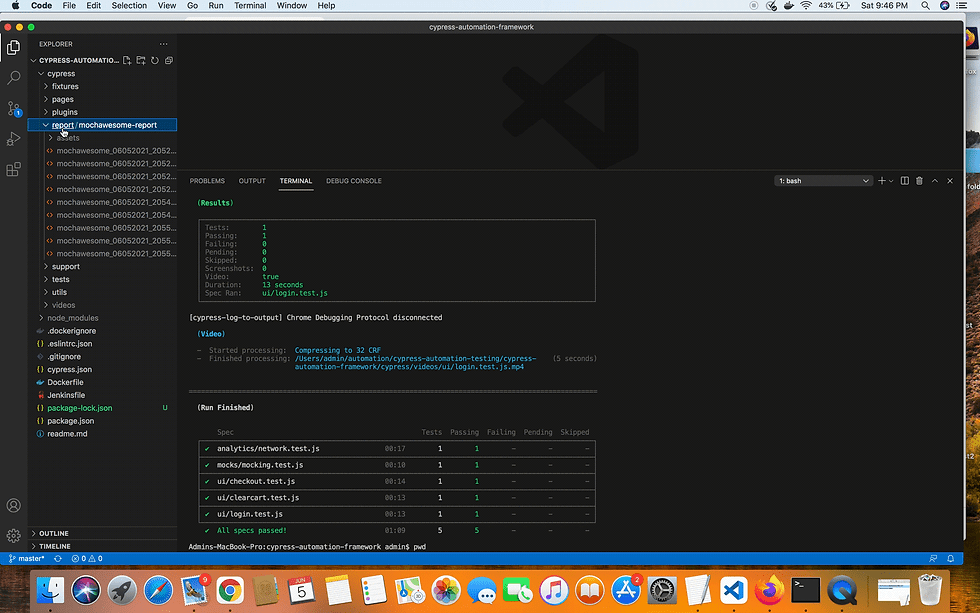
Conclusion
In this post, I’ve gone over how to write end-to-end UI tests and mocking test for one of react application https://shyedhu-react-app-3000.herokuapp.com/ using the Cypress.io
I hope you enjoyed on this post and helps you to get started with Cypress automation testing, and you will apply these approaches to your project !
If you have any questions please feel free to reach out to me on sayeedajeez55@gmail.com
Here is my repository that contains cypress automation framework code for reference purposes.
Happy Automation!!!
Kommentare