How to develop an API automation framework from scratch using python?
- Shyedhu
- Oct 19, 2021
- 2 min read
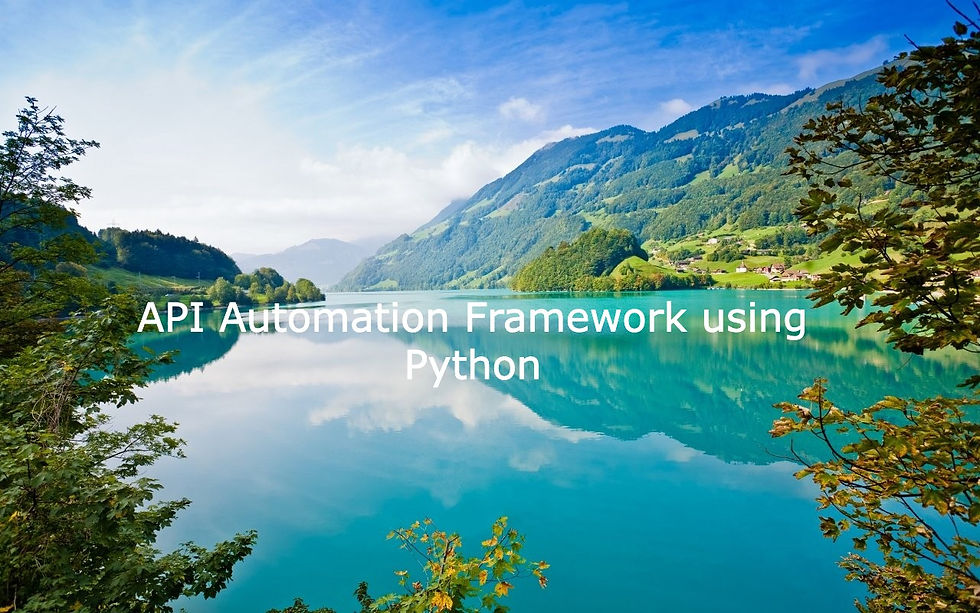
In this blog post, I’m going to show you how to develop an API automation framework from scratch using pytest with python. This blog post is writing some API tests against this API: https://nodes-api-demo.vercel.app/api/products
Prerequisites
Please ensure you have python3 installed on your machine. If not already installed, you can go to https://www.python.org/downloads/ and download the latest version of python for your OS and run the installer
Getting Started
Install the requests and pytest
pip install -U requests
We also need a testing framework to provide us with a test runner, I selected the pytest
pip install -U pytest
Clone this repo locally:
git clone https://github.com/shyedhu/pytest-automation-framework.git
To generate HTML reports with the Pytest framework we have to install a plugin. For the installation, we shall execute the command
pip install pytest-html
Writing API Test
Now, add a file name product_test.py under the tests folder and add the following statements to it:
import pytest
from helpers.utils import readdatas,getresponsetext
@pytest.mark.parametrize("name, price, available_quantity", readdatas())
def test_product_attribute(name, price, available_quantity,api_url):
products = getresponsetext(api_url)
for product in products:
if product["name"] == name:
assert product["price"] == int(price)
assert product["available_quantity"] == int(available_quantity)
Grouping Tests
In pytest we can group tests using markers on test functions.
Example code,
import pytest
from helpers.utils import getresponse
@pytest.mark.regression
def test_response_status_code(api_url):
response = getresponse(api_url)
print("response status_code ", response.status_code)
assert response.status_code == 200
@pytest.mark.regression
def test_response_headers_content(api_url):
response = getresponse(api_url)
print("response headers ", response.headers["Content-Type"])
assert response.headers["Content-Type"] == "application/json; charset=utf-8"
Running API test locally
navigate into the project folder
cd pytest-automation-framework
python3 -m pytest tests/ --html=report.html
Parallel testing
Parallel testing means we can execute tests by splitting the number of processors, called workers. We can assign multiple workers and execute.
Pytest runs all the test files in order. While executing a bunch of tests, it automatically increases execution time. In this scenario, parallel testing helps to run tests in parallel.
python3 -m pytest tests/ -n 3 --html=report.html
Reporting
Once we execute the test command, HTML or XML files can be seen in the project tree.
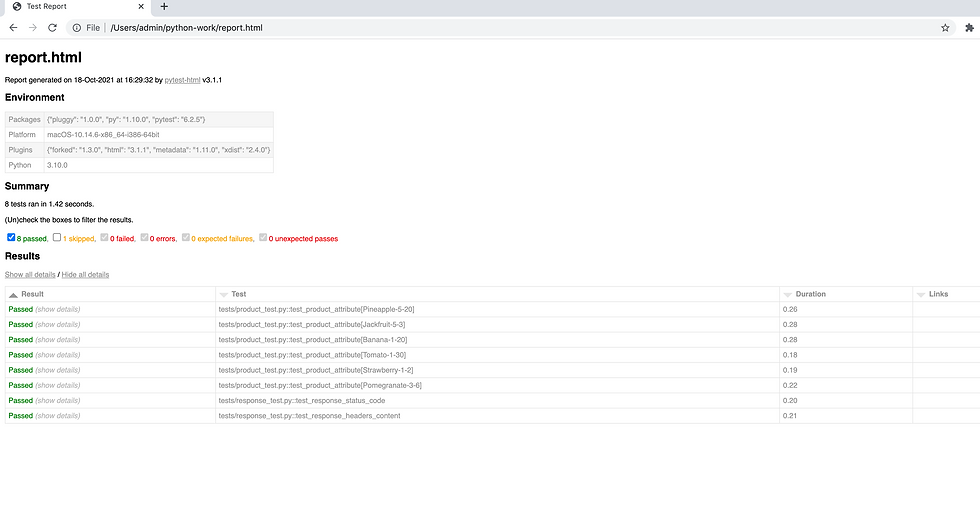
Conclusion
In this blog post, I’ve gone over how to develop an API automation framework from scratch using pytest with python for one of the API https://nodes-api-demo.vercel.app/api/products
I hope you enjoyed this post and helps you to get started with API automation testing, and you will apply these approaches to your project!
If you have any questions please feel free to reach out to me at sayeedajeez55@gmail.com
Here is my repository that contains an API Automation for reference purposes.
Comments