How to develop an e2e web UI automation framework from scratch using cypress.io with mock data?
- Shyedhu
- Jun 12, 2021
- 3 min read
Updated: Oct 15, 2021
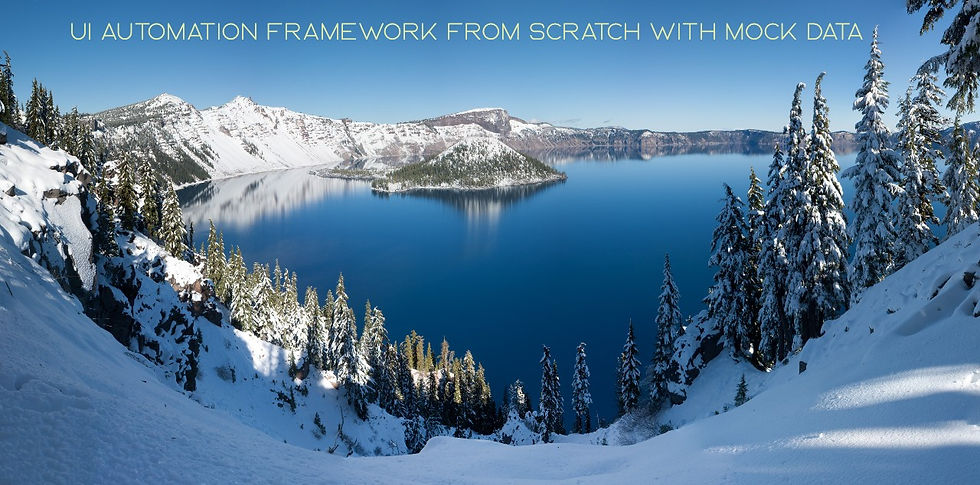
In this blog post, I’m going to show you how to develop an end-to-end web UI automation framework from scratch using cypress.io with mock data.
Prerequisites
Install Node.js (together with the NPM tool) instructions are available on https://nodejs.org/en/
Getting Started
Let’s start by creating a folder cypress-mocking. In your terminal run the following commands:
mkdir cypress-mocking
cd cypress-mocking
Now let’s add a package.json file to import all of the required dependencies
Add the following to the package.json file
{
"scripts": {
"cy:open": "./node_modules/.bin/cypress open",
"lint:run": "eslint '**/*.js'",
"lint:fix": "eslint --fix '**/*.js'"
},
"dependencies": {
"cypress": "7.4.0",
"ls": "^0.2.1",
"mocha": "^5.2.0",
"mochawesome": "^4.1.0",
"mochawesome-merge": "^4.0.0",
"rimraf": "^3.0.2",
"yargs": "^15.1.0"
},
"devDependencies": {
"cypress-log-to-output": "^1.0.8",
"eslint": "^7.27.0",
"eslint-config-airbnb-base": "^14.2.1",
"eslint-plugin-import": "^2.23.4"
}
}
Next, Let’s create a few subfolders in the root folder
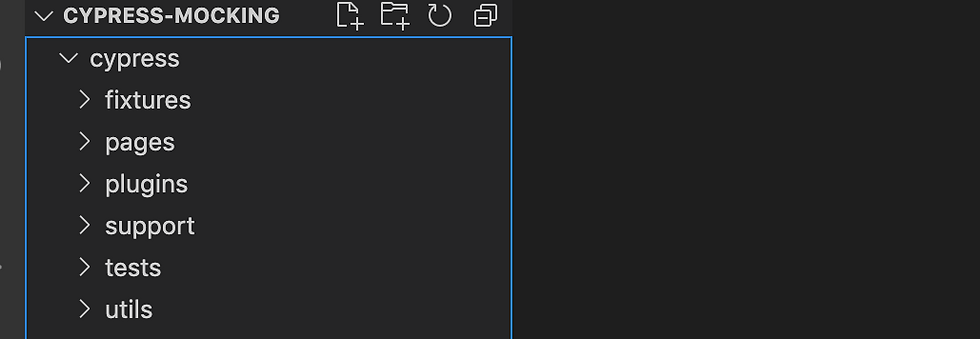
Next, Let’s add the cypress.json configuration file in the root folder, This JSON file is used to store any configuration values you supply.

Here's cypress mocking project structure

Next, Let's add products.json and users.json files in the fixtures folder, This is .json file should be in the fixtures folder as cypress will automatically search for test data inside this folder by default until and unless the path is specified otherwise.

Next, Let's see the Mock Data Approaches using cypress. Most web applications have a backend API that serves data. Setting up the data is essential to write more tests and is often a painful task. Let us explore different options to set up mock data for testing using cypress.
There are two ways we could be mocking, 1. Inline code Mocking 2. Fixtures
Let’s see mocking data using the Fixtures approach which is essentially the same as Inline code Mocking but instead of having the response as JavaScript code we point to a JSON file.
Make sure to add the following products.json in the fixtures folder
[
{
"id": 1,
"name": "Mango",
"image": "mango.png",
"available_quantity": 50,
"price": 7,
"description": "This is Mango"
}
]
we can now instruct cypress to use the above JSON file like so:
cy.fixture('products.json').as('productsData');
// mocking the products api response data
cy.route('GET', 'https://nodes-api-demo.vercel.app/api/products', '@productsData').as('productsapi');
Writing Mock Test
Now, add a file name mocking.test.js under the tests folder and add the following statements to it:
/**
* @description Test class to mock products api response data
* @author Shyedhu
*
*/
describe('Validate the mocking response', () => {
beforeEach(() => {
cy.fixture('users.json').as('usersData');
cy.fixture('products.json').as('productsData');
cy.server();
// mocking the products api response data
cy.route('GET', 'https://nodes-api-demo.vercel.app/api/products', '@productsData').as('productsapi');
custom.navigateTo(Cypress.env('ENV'));
});
it('test mocking response', () => {
cy.get('@usersData').then((usersData) => {
// validate the login
cy.login(usersData.username, usersData.pass);
cy.wait('@productsapi');
// Assert on XHR
cy.get('@productsapi').then((xhr) => {
// Assert status code
expect(xhr.status).to.eq(200);
// Assert request method
expect(xhr.method).to.eq('GET');
// Assert request url
expect(xhr.url).to.match(/\/products$/);
// Assert response Body
const response = xhr.responseBody;
expect(response[0]).to.have.property('available_quantity', 50);
expect(response[0]).to.have.property('price', 7);
expect(response[0]).to.have.property('description', 'This is Mango');
});
});
});
})
This is mocking test will let users navigate to http://shyedhu-shop-react-app.s3-website-us-west-2.amazonaws.com and validate the login flow and then validate the mocked API response data on the home page.
Running a mock test locally
First, Navigate into the project root folder and then install all dependencies using the following command. This will install all of the necessary packages to get cypress installed on your local machine.
npm install
Running a mock test on chrome browser
npm run cy:run:chrome
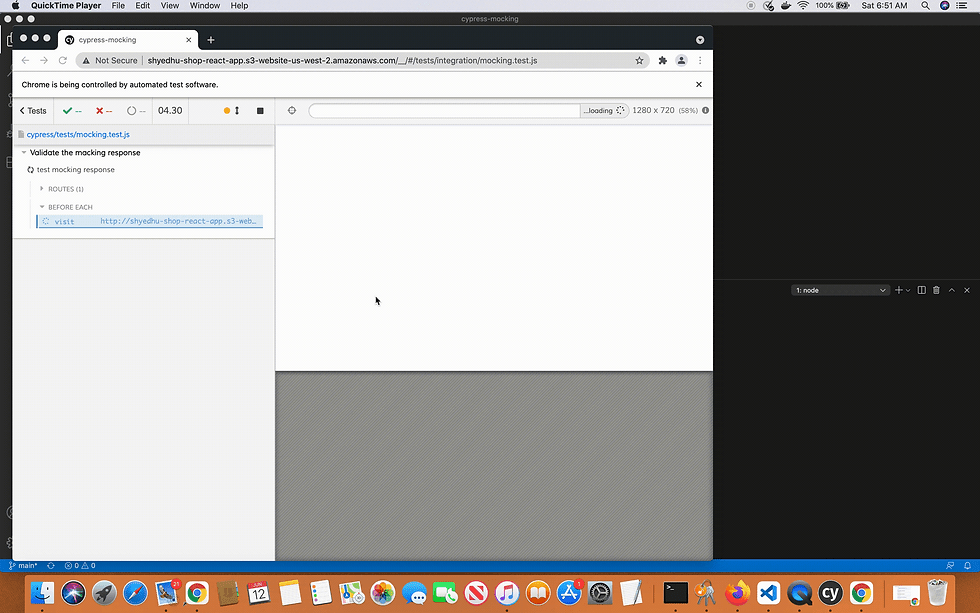
Conclusion
In this blog post, I’ve gone over how to develop an e2e web UI automation framework from scratch using cypress.io with mock data for one of react web application http://shyedhu-shop-react-app.s3-website-us-west-2.amazonaws.com
I hope you enjoyed this post and helps you to get started with Web UI automation testing, and you will apply these approaches to your project!
If you have any questions please feel free to reach out to me at sayeedajeez55@gmail.com
Here is my repository that contains an end-to-end web UI test for reference purposes.
Comments